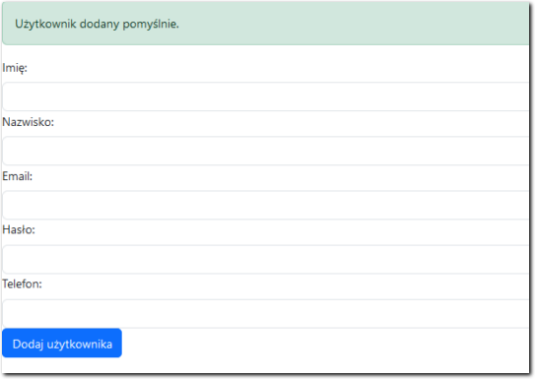
Kod po stronie frontend-u obsługujący dodanie użytkownika.
import "bootstrap/dist/css/bootstrap.min.css";
import React, { useState } from "react";
import { Form, Button, Alert } from "react-bootstrap";
const AddUser = ({ jwtToken }) => {
const [formData, setFormData] = useState({
name: "",
lastName: "",
email: "",
password: "",
phone: "",
});
const [error, setError] = useState(null);
const [success, setSuccess] = useState(null);
const handleChange = (e) => {
const { name, value } = e.target;
setFormData((prevData) => ({
...prevData,
[name]: value,
}));
};
const handleSubmit = async (e) => {
e.preventDefault();
try {
if (!jwtToken) {
setError("Brak autoryzacji. Zaloguj się.");
return;
}
const endpoint = "http://localhost:5203/api/Login/AddUser";
const response = await fetch(endpoint, {
method: "POST",
headers: {
"Content-Type": "application/json",
Authorization: `Bearer ${jwtToken}`,
},
body: JSON.stringify(formData),
});
if (response.ok) {
setSuccess("Użytkownik dodany pomyślnie.");
setError(null);
setFormData({
name: "",
lastName: "",
email: "",
password: "",
phone: "",
});
} else {
setError("Błąd dodawania użytkownika.");
setSuccess(null);
}
} catch (error) {
setError(`Błąd: ${error.message}`);
setSuccess(null);
}
};
return (
<Form onSubmit={handleSubmit}>
{error && <Alert variant="danger">{error}</Alert>}
{success && <Alert variant="success">{success}</Alert>}
<Form.Group controlId="formName">
<Form.Label>Imię:</Form.Label>
<Form.Control
type="text"
name="name"
value={formData.name}
onChange={handleChange}
required
/>
</Form.Group>
<Form.Group controlId="formLastName">
<Form.Label>Nazwisko:</Form.Label>
<Form.Control
type="text"
name="lastName"
value={formData.lastName}
onChange={handleChange}
required
/>
</Form.Group>
<Form.Group controlId="formEmail">
<Form.Label>Email:</Form.Label>
<Form.Control
type="text"
name="email"
value={formData.email}
onChange={handleChange}
required
/>
</Form.Group>
<Form.Group controlId="formPassword">
<Form.Label>Hasło:</Form.Label>
<Form.Control
type="password"
name="password"
value={formData.password}
onChange={handleChange}
required
/>
</Form.Group>
<Form.Group controlId="formPhone">
<Form.Label>Telefon:</Form.Label>
<Form.Control
type="text"
name="phone"
value={formData.phone}
onChange={handleChange}
required
/>
</Form.Group>
<Button variant="primary" type="submit">
Dodaj użytkownika
</Button>
</Form>
);
};
export default AddUser;
Endpoint obsługujący po stornie backend-u
[HttpPost("AddUser")]
[Authorize (Roles="User")]
public async Task<ActionResult<bool>> AddUser(UserDto userDto)
{
ValidationResult result = await _validator.ValidateAsync(userDto);
if (!result.IsValid)
{
return StatusCode(300,"Wrong Data");
}
return Ok(await _userServices.AddUser(userDto));
}
Usługa:
public async Task<bool> AddUser(UserDto userDto)
{
var result = _mapper.Map<User>(userDto);
result.Id = Guid.NewGuid();
result.DateCreate = DateTime.Now;
result.Password = _passwordHasher.HashPassword(result, userDto.Password);
await _contextModel.Users.AddAsync(result);
await _contextModel.SaveChangesAsync();
return true;
}
Walidacja (fluentValidation) :
public class UserValidation : AbstractValidator<UserDto>
{
public UserValidation()
{
RuleFor(x => x.Email).EmailAddress().NotNull().NotEmpty().WithMessage("Bad data or string is not email address!");
RuleFor(x => x.Password).MinimumLength(15).MaximumLength(32).NotNull()
.NotEmpty().WithMessage("Bad data ! or minimal char 15 and maximum length 32")
.Matches("[A-Z]").WithMessage("'{PropertyName}' must contain one or more capital letters.")
.Matches("[a-z]").WithMessage("'{PropertyName}' must contain one or more lowercase letters.")
.Matches(@"\d").WithMessage("'{PropertyName}' must contain one or more digits.")
.Matches(@"[""!@$%^&*(){}:;<>,.?/+\-_=|'[\]~\\]").WithMessage("' must contain one or more special characters.");
RuleFor(x => x.Phone).NotNull().NotEmpty().WithMessage("Bad data !");
RuleFor(x => x.LastName).NotNull().NotEmpty().WithMessage("Bad data !");
RuleFor(x => x.Name).NotNull().NotEmpty().WithMessage("Bad data !");
RuleFor(x => x.Phone).NotNull().NotEmpty().WithMessage("Bad data !");
}
}

Użytkownik dodany do bazy.